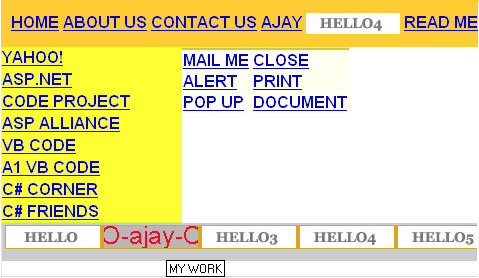
Introduction
There are various approaches for creating a header and footer for a site. Most of the time there is a mode of creating a static menu for header and footer. Time comes when looking at the same menu over and over again bores a user or at least the client. Why not give the client a menu where he can have fun and can make changes the way he wants?
There are almost countless methods of creating a dynamic menu and displaying it on our presentation layer. JavaScript, DHTML, XML and many other scripts come to our aid for creating a menu for header and footer. Being an ASP person, I have tried to create a menu using ASP.NET (C#) and XML that is easily understandable and handy too. I came across a header/footer sample on the net that allowed you to add the menu to your page without having to add any ASCX control. This inspired me to create this sample application that can do the thing without ASCX.
Using the code
Unzip the files to a folder named HeaderFooter. Create a virtual directory with your IIS named HeaderFooter. In your browser, open the page using the following address: http://localhost/HeaderFooter/index.aspx. You will find three class files that are responsible for this beautiful menu work.
ClsCreateHeader.cs
public System.Web.UI.WebControls.DataList dlheader;
private string xmlfile;
public DataList SetMenu(string menu)
{
ListProperties(menu);
return dlheader;
}
public void ListProperties(string menu)
{
dlheader = new System.Web.UI.WebControls.DataList();
dlheader.ItemTemplate = new DatalistLinkColumn();
dlheader.SeparatorTemplate = new DatalistSeparateColumn();
dlheader.RepeatDirection=RepeatDirection.Vertical;
dlheader.RepeatColumns=20;
dlheader.DataSource = GetMenu(menu);
dlheader.DataBind();
}
public DataView GetMenu(string xmltable)
{
DataSet dsMenu = new DataSet();
dsMenu.ReadXml
(System.Web.HttpContext.Current.Server.MapPath(GetConfigSettings()));
DataView dvfilterheader = new DataView(dsMenu.Tables[xmltable]);
dvfilterheader.RowFilter="bview=true";
dvfilterheader.Sort = "vworder ASC";
return dvfilterheader;
}
<configuration>
<appSettings>
<add key="xmlfile" value="XMenu.xml"></add>
</appSettings>
</configuration>
private string GetConfigSettings()
{
NameValueCollection config =
(NameValueCollection)ConfigurationSettings.GetConfig("appSettings");
xmlfile = config["xmlfile"];
return xmlfile;
}
DatalistLinkColumn.cs
public void InstantiateIn(Control container)
{
HyperLink hlheader = new HyperLink();
hlheader.DataBinding += new EventHandler(this.BindLinkColumn);
container.Controls.Add(hlheader);
}
public void BindLinkColumn(object sender, EventArgs e)
{
HyperLink hl = (HyperLink)sender;
DataListItem container = (DataListItem)hl.NamingContainer ;
"vwtext"));
String strVals =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwtext"));
hl.Text = strVals;
if(Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwas"))=="txt")
hl.Text =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwtext"));
if(Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwas"))=="img")
hl.ImageUrl =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwimage"));
if(Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwoverimage")) != "")
{
hl.Attributes[ "onMouseOver" ] = "this.firstChild.src = '"
+ Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwoverimage")) + "'";
hl.Attributes[ "onMouseOut" ] = "this.firstChild.src = '"
+ Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwimage")) + "'";
}
hl.NavigateUrl =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"url"));
hl.ToolTip =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"tooltip"));
hl.CssClass =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"class"));
hl.Target =
Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"target"));
}
DatalistSeparateColumn.cs
public void InstantiateIn(Control container)
{
HyperLink hlseparate = new HyperLink();
hlseparate.DataBinding += new EventHandler(this.BindSeparateColumn);
container.Controls.Add(hlseparate);
}
public void BindSeparateColumn(object sender, EventArgs e)
{
HyperLink hl = (HyperLink)sender;
DataListItem container = (DataListItem)hl.NamingContainer ;
SeparatorText = "";
hl.Text = SeparatorText;
}
Both the above classes use ITemplate
interface to create controls within Datalist
’s ItemTemplate
and SeparateItemTemplate
. ITemplate
defines the method to implement population of an ASP.NET server control with child controls when using a control with inline templates declared in an .aspx file.
index.aspx.cs
What you need here is a placeholder to show your menu:
private ClsCreateHeader clsch = new ClsCreateHeader();
private void Page_Load(object sender, System.EventArgs e)
{
phheader.Controls.Clear();
phheader.Controls.Add(clsch.SetMenu("Header"));
DataList dlmenu = new DataList();
dlmenu = clsch.SetMenu("Header");
dlmenu.BackColor=Color.Goldenrod;
dlmenu.RepeatColumns=10;
phheader.Controls.Add(dlmenu);
}
In the sample index.aspx file, four different menus are shown:
- Header – You will find simple straightforward links to different pages. One thing to notice here is the use of a text link and an image link in the same menu.
- LeftBar – The menu is set to popup different web sites (not misused … just promoting them).
- RightBar – Displays use of various JavaScript functions.
- Footer – Use of web controls loading into the index file.
Xmenu.xml
<Menu>
<Header
id="1" –a simple id for numbering the items
vwtext="HOME" –text to be displayed.
vworder="1" –view order default set to ascending. Can be altered
bview="true" –want to view or hide this item?
vwimage="images/hello.jpg" –alternative for text (good hmm)
vwoverimage ="images/mouseover.jpg" –this will be your mouseover image
target="_self" –must be set. _self, _blank, _top
url="index.aspx" –url for the link
vwas="txt" –can be "txt" or "img"
(though vwtext and vwimage values are inserted,
this will set how to view the link)
class="A" //cssheader.css file class
tooltip="HOME PAGE" –tool tip to be viewed with link
></Header>
</Menu>
Web.Config
This is important because you can also add your own XML file (not altered with the attributes as in XMenu.xml).
<appSettings>
< add key ="xmlfile" value ="XMenu.xml"></ add>
</appSettings>
Points of interest
A small but good use of swapping images. Check the footer hyperlinks and find the code in DatalistLinkColumn.cs. I rarely found an article on net about this. I admit I have used one of the help forums to complete this particular task.
hl.Attributes[ "onMouseOver" ] = "this.firstChild.src =
'" + Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwoverimage")) + "'";
hl.Attributes[ "onMouseOut" ] = "this.firstChild.src =
'" + Convert.ToString(DataBinder.Eval(((DataListItem)container).DataItem,
"vwimage")) + "'";
But I am still unaware about the use of this.firstChild.src
and how this works. An important point to note here. For the image control, the use of this.src
will work fine whereas this is not the case with hyperlink.
He is a Professional developer and project leader, working on .Net Technologies. He works in a firm based in the Hub of IT - Bangalore. His core interests are C#, ASP.net and any microsoft technology that brings him near to Web. Likes to share his views and ideas that help other developers.